flask中跳转的同时设置cookie
最近在使用flask的时候,有一个比较麻烦的事情,在跳转网页的时候需要设置cookie,使用单独设置cookie与单独跳转都比较简单,
跳转网页
| from flask import redirect
@app.route("/redirect")
def redirecttest():
return redirect("/test")
|
设置cookie
| from flask import Flask,make_response
app = Flask(__name__)
@app.route("/set_cookie")
def set_cookie():
#先创建响应对象
resp = make_response("set cookie test")
# 设置cookie cookie名 cookie值 默认临时cookie浏览器关闭即失效
# 通过max_age控制cookie有效期, 单位:秒
resp.set_cookie("display","yangyanxing",max_age=3600)
return resp
|
可以看到,设置cookie的response
最后是通过return 回来的,但是上面的网页跳转则是通过redirect()
函数返回的,所以这两个没法同时的使用。
查看redirect()
的源码
| def redirect(location, code=302, Response=None):
if Response is None:
from .wrappers import Response
display_location = escape(location)
if isinstance(location, text_type):
# Safe conversion is necessary here as we might redirect
# to a broken URI scheme (for instance itms-services).
from .urls import iri_to_uri
location = iri_to_uri(location, safe_conversion=True)
response = Response(
'<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 3.2 Final//EN">\n'
"<title>Redirecting...</title>\n"
"<h1>Redirecting...</h1>\n"
"<p>You should be redirected automatically to target URL: "
'<a href="%s">%s</a>. If not click the link.'
% (escape(location), display_location),
code,
mimetype="text/html",
)
response.headers["Location"] = location
return response
|
可以看到,其实redirect函数也是通过构造一个response最后再将这个resonse return 出去,所以我们是否可以构造一个类似于redirect函数中的response对象,然后设置好status code是不是就可以了呢?
| from flask import Flask,make_response,escape
app = Flask(__name__)
@app.route("/test")
def test():
response = make_response(
'<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 3.2 Final//EN">\n'
"<title>Redirecting...</title>\n"
"<h1>Redirecting...</h1>\n"
"<p>You should be redirected automatically to target URL: "
'<a href="%s">%s</a>. If not click the link.'
% (escape("https://www.baidu.com"),
escape("https://www.baidu.com")), 302)
response.set_cookie('display',"yangyanxing")
return respose
|
运行上面的代码,发现停留在/test
页面,显示上面的make_response
的文字,并没有跳转,但是cookie中已经有display的cookie了,说明设置cookie成功了,跳转也成功了一半,只是它没有真正的跳转,还需要在网页上点击一下。
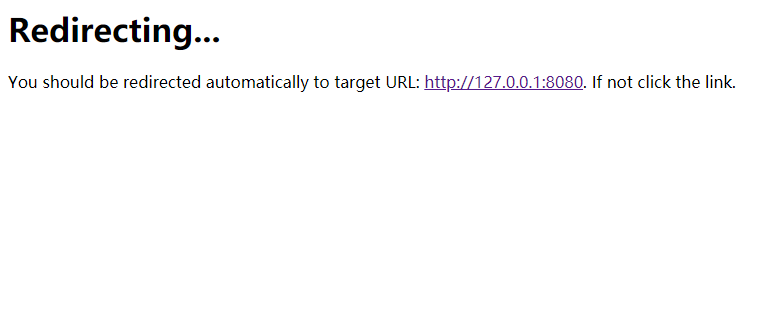
redirect函数中是通过 response.headers["Location"] = location
来设置的,在make_response
的返回响应对象中是通过response.location = 'xxxx'
来设置的
| from flask import Flask,make_response,escape
app = Flask(__name__)
@app.route("/test")
def test():
response = make_response(
'<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 3.2 Final//EN">\n'
"<title>Redirecting...</title>\n"
"<h1>Redirecting...</h1>\n"
"<p>You should be redirected automatically to target URL: "
'<a href="%s">%s</a>. If not click the link.'
% (escape("https://www.baidu.com"),
escape("https://www.baidu.com")), 302)
response.set_cookie('display',"yangyanxing")
`response.location = escape("https://www.baidu.com")
return respose
|
这时就可以正常的跳转了